How to run a Python script
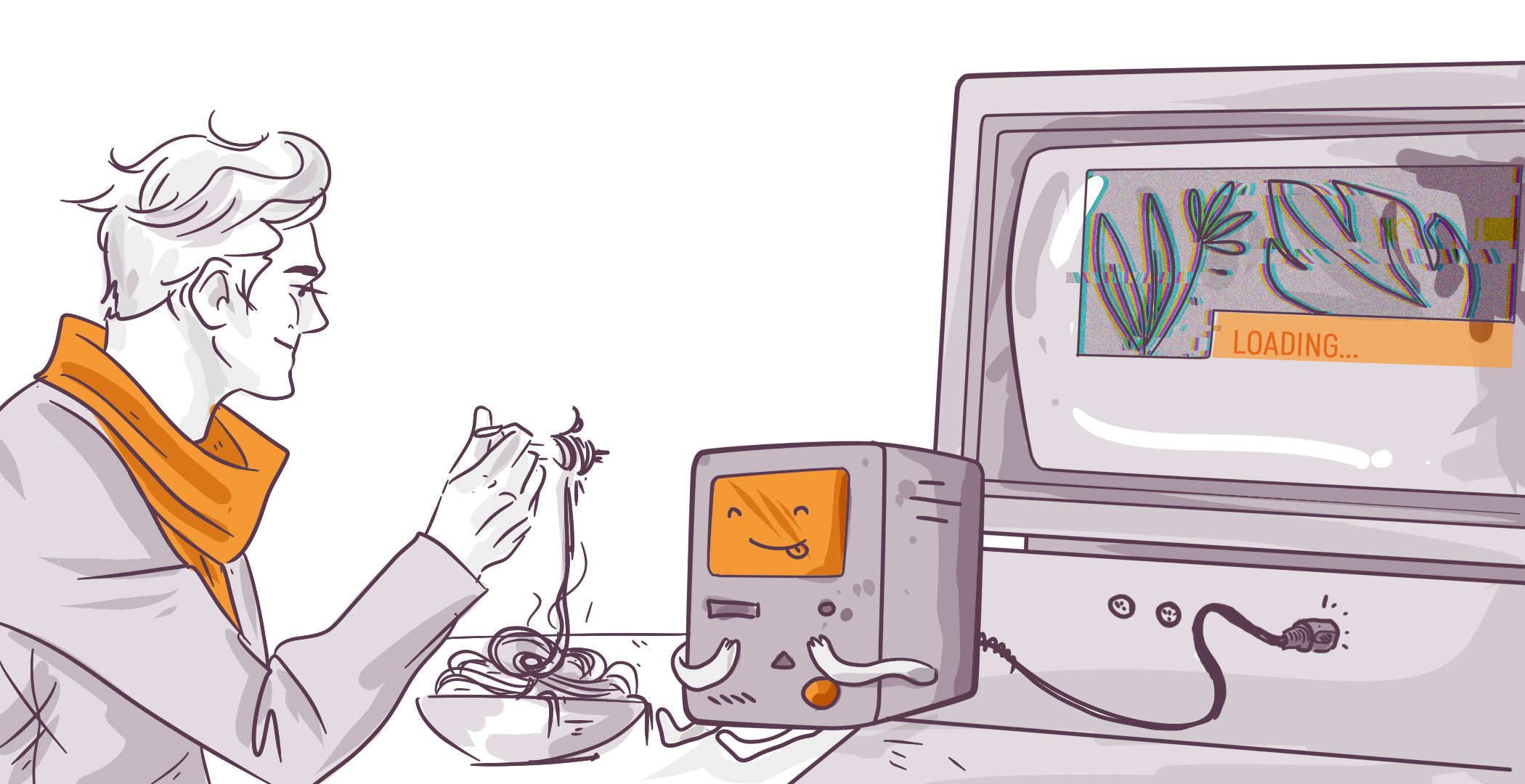
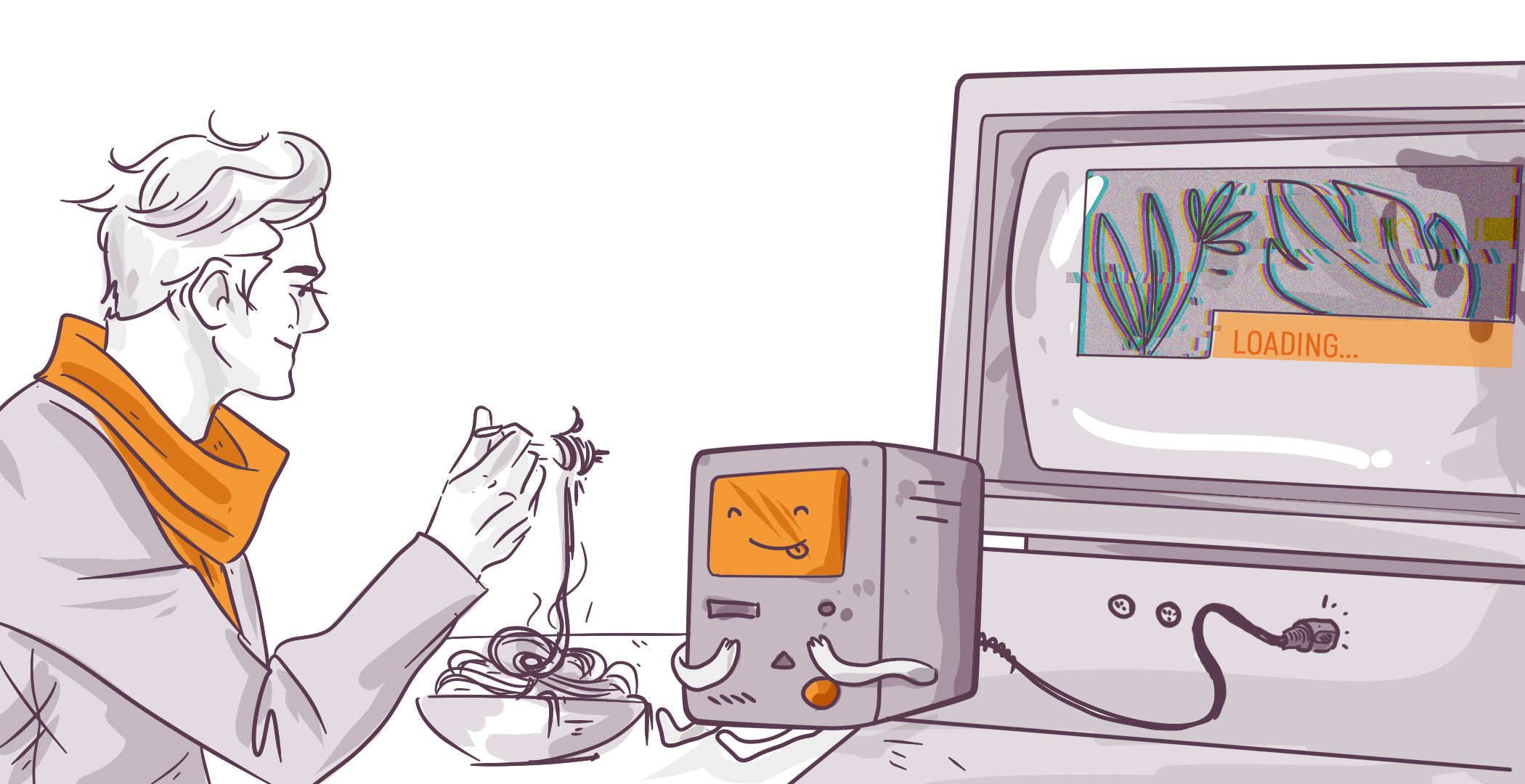
If you are a beginner Python developer, besides syntax and basic operators usage rules, you also need to know how to run your code. This is the only thing that lets you understand if your script actually works or has any errors. Let’s have a more detailed look at how to run scripts in OS command line, integrated development environment or just OS interface. This might help you choose the best option and make your work more effective.
Python interpreter
The language we’re talking about is one of the most advanced languages thus far. It allows fast and effective solving of different problems in different fields. However, the term ‘Python’ also includes the interpreter, a computer program which runs the scripts you wrote. This program is an additional software layer between the hardware of your PC and your code.
There are several types of interpreters:
- written in С;
- written in Java;
- written in Python;
- implemented in .NET. environment.
Your choice doesn’t affect the end user anyhow. No matter what’s been chosen, the code will be executed according to the rules of the chosen language.
Your program can be run as a ready-to-use program sequence (script or module) or as separate code pieces which are entered right into the program window.
Interactive mode
You can use the interpreter in the interactive mode to test some commands. To do this you need to go to the command line of your OS and enter the command which runs the interpreter.
This is how it looks for Linux:
$ python3
Python 3.6.7 (default, Oct 22 2018, 11:32:17)
[GCC 8.2.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
Now you can enter the commands which will be executed afterwards. The only disadvantage it has is that the sequence you’ve entered won’t be saved if you close the current session.
Interactive code execution is good when you need to immediately test some fragment of the code. Moreover, while you’re trying to find your way around Python, you can use it to test some operators’ actions on the fly. It’s awesome because it lets you have a look at the functions you’re interested in without wasting your time on writing single-purpose scripts.
You can exit the interactive mode with quit() command or by closing the Windows command line window.
Before launching the interpreter itself, to open the terminal or command line you need to make several steps, such as:
- in Windows press Win and R, enter ‘cmd’ and select ‘Ok’.
- in Linux or other similar OSs download a special program, such as xterm or Konsole, to get access to the command line.
- in macOS select ‘Applications’, then ‘Utilities’ and then ‘Terminal’ to get access to the console.
How the Python interpreter for scripts works
The execution of the written scripts and modules is done in a batch and is implemented according to a complex procedure including these steps:
Consecutive processing of all the operators written in the script.
Compiling the source code into an intermediate format. The interpreter creates byte-code, a low-level programming language suitable for any platform or OS. You need it to optimize the process of executing the script.
Executing the compiled code. At this point, the Python Virtual Machine (PVM) comes into action and starts iterating each operator from the script and running it, as if you were entering each command one by one in the interactive interpreter.
Script running in the command line
You can enter and execute any number of code lines using the aforementioned interactive mode. But when you close the window, they are deleted. That’s why real Python programs are made as scripts and look like simple text files. These files are given extensions .py or .piw., so you wouldn’t confuse them with other text files.
You can create text files using whatever text editor you like, e.g. Notepad. However, more advanced solutions, e.g. Sublime Text, are way better. Let’s try to make an easy sample script, which is usually used to get the gist of some programming language.
#!/usr/bin/env python3
print('Hello World!')
You can store the file in your work folder with any name and .py. extension.
To run the script you need to use the programming language interpreter and specify the name of the created file as an additional parameter.
$ python3 hello.py
Hello World!
In the example above the file was called ‘hello.py’. After entering the command you need to press ‘Enter’ and see the result of the script on the screen, which is a classic ‘Hello, World’ phrase.
If the file with a program hasn’t been saved to the folder with the interpreter, you need to specify the path to it.
Reassign the output device
When executing Python code you sometimes need to save the results showed on the screen. They are later used to analyze and search for mistakes and other things. If you need it, you should use this command:
$ python3 hello.py > output.txt
The script creates the file called output.txt, where everything that should have appeared on the screen while you were working on the program would be saved. This is the standard syntax provided by the OS.
If there’s no file with such a name, the OS will create it automatically. If there is one, its data will be rewritten and the previous data will be deleted. If you want to add data to the end of the text file, you need to enter >> instead of >.
Running from a command line using an interpreter
In the latest Windows versions, you can run Python scripts without entering the name of the interpreter in the command line. You just need to enter the file name with its extension.
C:\devspace> hello.py
Hello World!
This is because when you click on a file or run it from the command line, your OS automatically starts searching for an associated program and runs it too. You open the files by clicking on them in the same way.
You run the scripts in Unix the same way. However, to do that you need to add #!/Usr/bin/env python to the first line of the text file with the commands. This text indicates the program that makes the launch.
Script running in an interactive mode
In the interactive mode described earlier, the user can load the file with the already written command sequence and run it. This option can be used when the module contains function, method and other operator calls that output the text to the screen. Otherwise, you won’t be able to see the results.
You can run the script in the interactive mode with this command:
>>> import hello
Hello World!
Please note that this command can be triggered once during one interactive session. So if you make changes to the file and restart the script with this command, nothing will happen.
Conclusion
Now you know that Python commands and scripts can be run in different ways and in different modes. Knowing all that, you can pick up the best option needed for some specific task, make your work faster, more productive and flexible.